1 min to read
Tune Hyperparameter
Tune the hyperparameter with the help of A.I.
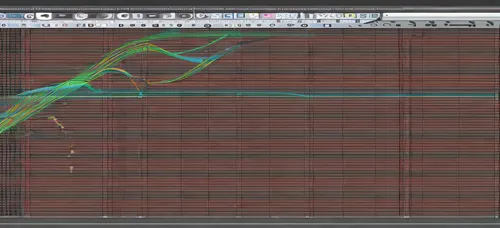
Prompt
I want you to act as a data scientist and code for me. I have trained a [model name]. Please write the code to tune the hyper parameters.
Example answer
- Set the number of iterations: model.set_params(n_iter = [number])
- Set the maximum depth of each tree: model.set_params(max_depth=[number])
- Set the minimum information gain for each split: model.set_params(min_impurity_decrease=[number])
- Set the minimum number of samples required to split a node: model.set_params(min_samples_split=[number])
- Set the minimum number of samples required at each leaf node: model.set_params(min_samples_leaf=[number])
- Set the maximum features to consider when looking for the best split: model.set_params(max_features=[number])
#Import the relevant libraries
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from [model_name] import [model_name]
#Define the model
model = [model_name]()
#Define the parameters to tune
params = {'parameter_1':[0.2,0.4,0.6,0.8],
'parameter_2':[5, 10, 15, 20],
'parameter_3':[10, 15, 20, 25]
}
#Train model using grid search
from sklearn.model_selection import GridSearchCV
clf = GridSearchCV(model, params, cv=3, scoring='accuracy')
clf.fit(X, y)
#Display the best parameters
print("The best parameters are: " + str(clf.best_params_))
Comments