1 min to read
Train Classification Model
Build a ML model that predicts something
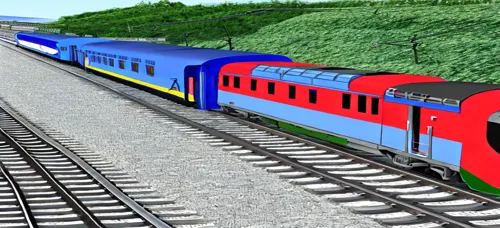
Prompt
I want you to act as a data scientist and code for me in python. I have a dataset of [describe dataset]. Please build a machine learning model that predict [target variable].
Example answer
- Step 1. Load the dataset into a pandas dataframe.
- Step 2. Preprocess the data, including data cleaning and feature engineering.
- Step 3. Split the data into training and test sets.
- Step 4. Select a machine learning model and train it on the training set.
- Step 5. Evaluate the model on the test set.
- Step 6. Make predictions with the trained model on new data.
Below is an example of how I would code this in Python:
# import libraries
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
# read the dataset
data = pd.read_csv('dataset.csv')
# split into features and target
X = data.drop('target_variable', axis=1)
y = data['target_variable']
# split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# scale the data
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# build the model
model = LogisticRegression()
model.fit(X_train_scaled, y_train)
# make predictions on the test set
y_pred = model.predict(X_test_scaled)
Comments